Overview of how to generate a dynamic PDF File
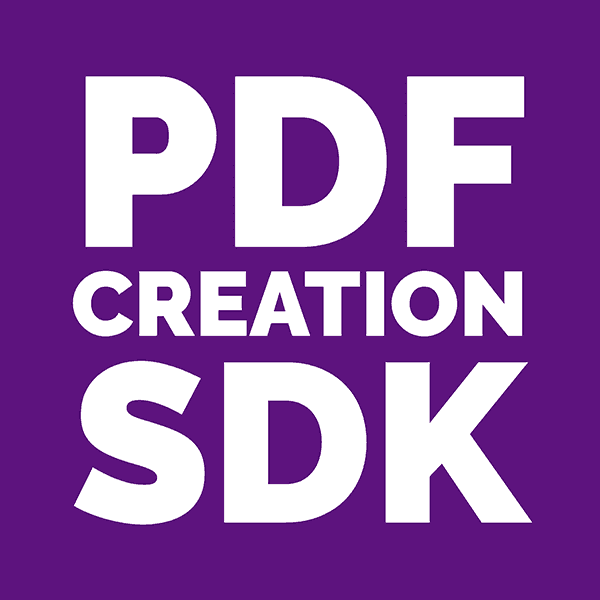
Creating PDFs programmatically from scratch using Visual Integrity’s PDF Creation SDK is simple. The Crete PDF File API is robust and covers the full spectrum of PDF 2.0 features including images, text strings and labels, form fields, compound objects, layers, line objects, polygons, tooltips and URLs, to name a few. With the PDF Creation API, split, combine, merge, add page numbers, and more.
This example shows how to generate dynamic PDF reports and statements using the API. It’s ideal for applications and services which require PDF output and data-driven PDF creation. Using simple language, create a PDF without any intermediate steps.
Here’s what programmatic PDF creation looks like:
// Create new PDF-file
Vg2Pdf_CreateFile(&pdf, filename, 0);
// Create layers
Vg2Pdf_CreateLayer(&layer1, pdf, “Layer One”, 1);
Vg2Pdf_CreateLayer(&layer2, pdf, “Layer Two”, 1);
// Create new page
Vg2Pdf_BeginPage(pdf, 612.0, 792.0);
// Add a line object to the page
Vg2Pdf_SetStrokeColor(pdf, 128, 0, 255);
Vg2Pdf_SetLineWidth(pdf, 3.0);
pt1.x = 100.0; pt1.y = 600.0;
pt2.x = 200.0; pt2.y = 450.0;
Vg2Pdf_DrawLine(pdf, &pt1, &pt2);
// Enter layer
Vg2Pdf_OpenLayer(pdf, layer1);
// Add text object to the page
Vg2Pdf_SetTextFont(pdf, “Calibri”, 0, 12.0);
Vg2Pdf_DrawText(pdf, 100.0, 100.0, 0.0, “Hello World”);
// Close layer
Vg2Pdf_CloseLayer(pdf, layer1);
// Add polygon to the page
Vg2Pdf_DrawPoly(pdf, &irpoly, 1);
// Add circle to the page
Vg2Pdf_DrawCircle(pdf, &cucircle);
// Add bezier objectto the page
Vg2Pdf_DrawPath(pdf, &cupath);
// Add image to the page
Vg2Pdf_DrawJpgImage(pdf, “hello.jpg”, pts);
// Add tooltip to the page
Vg2Pdf_CreateToolTip(pdf, 1, bbox, “TestToolTip”, “Hello World”);
// Add URL link to the page
Vg2Pdf_CreateURLLink(pdf, 1, bbox, 10.0, 0, “http://www.google.com”);
// End page
Vg2Pdf_EndPage(pdf);
// Create 2nd page
Vg2Pdf_BeginPage(pdf, 612.0, 792.0);
// Create link
Vg2Pdf_CreateLink(pdf, 2, bbox, 3, 0, 1, 792.0); /* from page 1 to page 2 */
// Add arc object to page
Vg2Pdf_DrawArc(pdf, 200.0, 300.0, 50.0, 0.0, 270.0);
// End page
Vg2Pdf_EndPage(pdf);
// Close the newly created PDF file
Vg2Pdf_CloseFile(pdf);